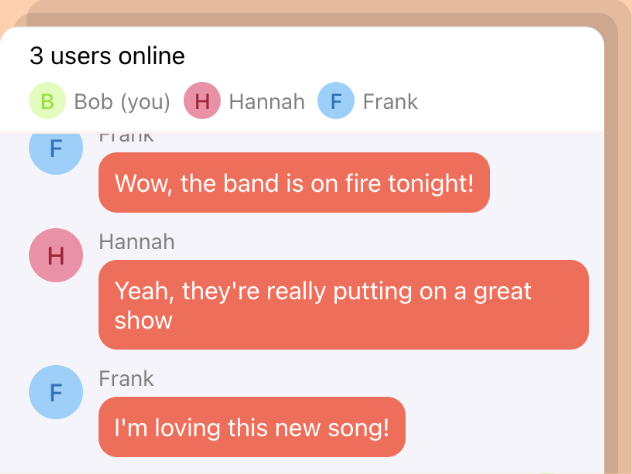
Android Chat Tutorial: Building A Realtime Messaging App
In this tutorial, we'll create a real-time group chat for Android using the Scaledrone Java API Client. The functionality will closely resemble that of popular messaging apps like WhatsApp, Facebook Messenger, and Signal.
You can find the complete source code on GitHub.
This tutorial will teach you:
- How to build a fully functional group chat.
- Designing the UI elements such as chat bubbles and text inputs.
- How to use Scaledrone as the realtime backend of your app.
The project might seem daunting at first, but the messaging code outside of the layout files is pretty short.
Setting up the project
Start by creating a new Android project. We are using Android Studio, but this tutorial will work with any IDE of choice.
Import the Scaledrone module
To add the Scaledrone dependency to your app, you need to add it to your build.gradle
file.
dependencies {
//...
compile 'com.scaledrone:scaledrone-java:0.6.0'
}
For Android to allow us to connect to the internet, we need to add the internet permission to the manifests/AndroidManifest.xml
file:
<!-- put this after the starting <manifest> tag and before the starting <application> tag -->
<uses-permission android:name="android.permission.INTERNET" />
Defining the UI layout
To start with the UI layout let's build the empty state. It consists of:
An empty ListView
into where the messages will go
An EditText
where the user can type their message
And finally, an ImageButton
as a button to send the message
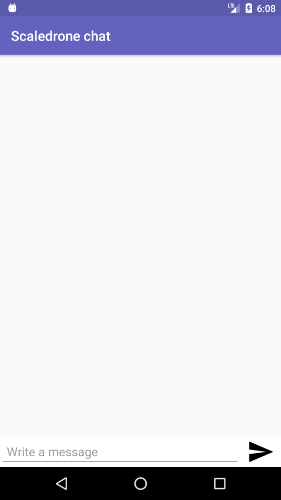
The base layout is defined in /res/layout/activity_main.xml
:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:clipToPadding="false"
android:focusableInTouchMode="true"
tools:context="com.example.scaledrone.chat.MainActivity">
<ListView
android:layout_width="match_parent"
android:id="@+id/messages_view"
android:layout_weight="2"
android:divider="#fff"
android:layout_height="wrap_content"
/>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="#fff"
android:orientation="horizontal">
<EditText
android:id="@+id/editText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="2"
android:ems="10"
android:hint="Write a message"
android:inputType="text"
android:paddingHorizontal="10dp"
android:text="" />
<ImageButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:scaleType="fitCenter"
android:padding="20dp"
android:layout_marginHorizontal="10dp"
android:background="@drawable/ic_send_black_24dp"/>
</LinearLayout>
</LinearLayout>
String constants are defined in /res/layout/activity_main.xml
:
<resources>
<string name="app_name">Scaledrone chat</string>
<string name="input_placeholder">Write a message</string>
</resources>
The icon for the send button is defined in /res/drawable/ic_send_black_24dp.xml
:
<vector xmlns:android="http://schemas.android.com/apk/res/android"
android:width="24dp"
android:height="24dp"
android:viewportWidth="24.0"
android:viewportHeight="24.0">
<path
android:fillColor="#FF000000"
android:pathData="M2.01,21L23,12 2.01,3 2,10l15,2 -15,2z"/>
</vector>
Next up, chat bubbles!
Our chat app is going to have two type of chat bubbles: a bubble for messages sent by us and bubbles for messages sent by others.
Chat bubble sent by us
The messages sent by us will look dark and be aligned to the right. We're using a drawable to get the border radius effect.
/res/drawable/my_message.xml
:
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<solid android:color="@color/colorPrimary" />
<corners android:topRightRadius="5dp" android:radius="15dp" />
</shape>
The message itself is just a simple TextView
aligned to the right.
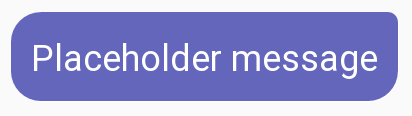
/res/layout/my_message.xml
:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:paddingVertical="10dp"
android:paddingRight="15dp"
android:paddingLeft="60dp"
android:clipToPadding="false">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/message_body"
android:background="@drawable/my_message"
android:textColor="#fff"
android:padding="10dp"
android:elevation="2dp"
android:textSize="18dp"
android:layout_alignParentRight="true"
android:text="Placeholder message"
/>
</RelativeLayout>
Chat bubble sent by others
The chat bubble sent by others within the group chat will be light and aligned to the left. In addition to the bubble itself, we will show an avatar (as a simple full-color circle) and the name of the user.
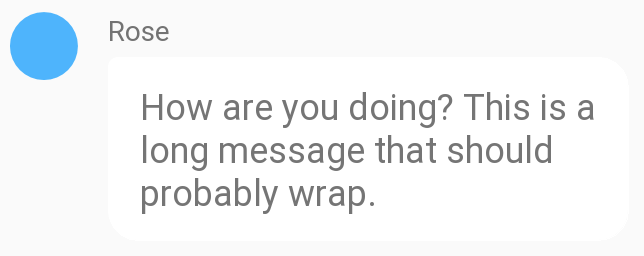
For the avatar let's define a circle shape under /res/drawable/circle.xml
:
<shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="oval">
<solid android:color="#48b3ff"/>
</shape>
And for the bubble let's create a shape with curved corners and the sharp corner on the left. This goes in /res/drawable/their_message.xml
:
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<solid android:color="#fff" />
<corners android:topLeftRadius="5dp" android:radius="15dp" />
</shape>
Putting it together their message bubble layout under /res/layout/their_message.xml
will look like this:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:paddingVertical="10dp"
android:paddingLeft="15dp"
android:paddingRight="60dp"
android:clipToPadding="false">
<View
android:id="@+id/avatar"
android:layout_alignParentLeft="true"
android:scaleType="centerInside"
android:background="@drawable/circle"
android:layout_width="34dp"
android:layout_height="34dp" />
<TextView
android:id="@+id/name"
android:layout_marginLeft="15dp"
android:layout_toRightOf="@+id/avatar"
android:layout_alignTop="@+id/avatar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:paddingBottom="4dp"
android:text="Rose"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/message_body"
android:layout_below="@+id/name"
android:layout_alignLeft="@+id/name"
android:background="@drawable/their_message"
android:paddingVertical="12dp"
android:paddingHorizontal="16dp"
android:elevation="2dp"
android:textSize="18dp"
android:text="How are you doing? This is a long message that should probably wrap."
/>
</RelativeLayout>
Hooking up the realtime messaging logic
We're finally done with the layout and can get to the interesting part!
Let's find the EditText
view from our layout and extend Scaledrone's RoomListener
so we could receive messages. Most of the methods will have minimal code in them, and we'll fill them up as the tutorial goes along.
public class MainActivity extends AppCompatActivity implements
RoomListener {
private String channelID = "CHANNEL_ID_FROM_YOUR_SCALEDRONE_DASHBOARD";
private String roomName = "observable-room";
private EditText editText;
private Scaledrone scaledrone;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// This is where we write the mesage
editText = (EditText) findViewById(R.id.editText);
}
// Successfully connected to Scaledrone room
@Override
public void onOpen(Room room) {
System.out.println("Conneted to room");
}
// Connecting to Scaledrone room failed
@Override
public void onOpenFailure(Room room, Exception ex) {
System.err.println(ex);
}
// Received a message from Scaledrone room
@Override
public void onMessage(Room room, com.scaledrone.lib.Message receivedMessage) {
// TODO
}
}
Connecting to Scaledrone
If you don't have a Scaledrone account yet, open up Scaledrone.com and create a new free account. To successfully connect to Scaledrone you need to get your own channel ID from the Scaledrone's dashboard. To do that go to the dashboard and click the big green +Create Channel button to get started. You can choose Never require authentication for now. Copy the channel ID from the just created channel and replace CHANNEL_ID_FROM_YOUR_SCALEDRONE_DASHBOARD
with it.
Connecting to Scaledrone can happen within the onCreate()
method, right after we have set up the UI. Scaledrone gives us the ability to attach arbitrary data to a user (users are called members in Scaledrone lingo), we're going to be adding a random name and color.
MemberData data = new MemberData(getRandomName(), getRandomColor());
scaledrone = new Scaledrone(channelID, data);
scaledrone.connect(new Listener() {
@Override
public void onOpen() {
System.out.println("Scaledrone connection open");
// Since the MainActivity itself already implement RoomListener we can pass it as a target
scaledrone.subscribe(roomName, MainActivity.this);
}
@Override
public void onOpenFailure(Exception ex) {
System.err.println(ex);
}
@Override
public void onFailure(Exception ex) {
System.err.println(ex);
}
@Override
public void onClosed(String reason) {
System.err.println(reason);
}
});
You might have noticed that we named our name Scaledrone room observable-room. You can name the room anything you want, a single user can actually connect to an infinite amount of rooms to provider for all sorts of application scenarios. However in order for messages to contain the info of the sender the room name needs to be prefixed with observable-. Read more..
To create the MemberData let's implement the getRandomName()
and getRandomColor()
functions as well as the MemberData
class itself.
For the sake of keeping this tutorial simple, we'll define a random username on the client side of the application. Later you can add fancy login functionality to your app. To create a random name, we pre-define two lists of random adjectives and nouns, then combine them randomly.
The random color function will be generating a random seven-character color hex such as #FF0000
.
public class MainActivity extends AppCompatActivity implements RoomListener {
/* ...
more code
... */
private String getRandomName() {
String[] adjs = {"autumn", "hidden", "bitter", "misty", "silent", "empty", "dry", "dark", "summer", "icy", "delicate", "quiet", "white", "cool", "spring", "winter", "patient", "twilight", "dawn", "crimson", "wispy", "weathered", "blue", "billowing", "broken", "cold", "damp", "falling", "frosty", "green", "long", "late", "lingering", "bold", "little", "morning", "muddy", "old", "red", "rough", "still", "small", "sparkling", "throbbing", "shy", "wandering", "withered", "wild", "black", "young", "holy", "solitary", "fragrant", "aged", "snowy", "proud", "floral", "restless", "divine", "polished", "ancient", "purple", "lively", "nameless"};
String[] nouns = {"waterfall", "river", "breeze", "moon", "rain", "wind", "sea", "morning", "snow", "lake", "sunset", "pine", "shadow", "leaf", "dawn", "glitter", "forest", "hill", "cloud", "meadow", "sun", "glade", "bird", "brook", "butterfly", "bush", "dew", "dust", "field", "fire", "flower", "firefly", "feather", "grass", "haze", "mountain", "night", "pond", "darkness", "snowflake", "silence", "sound", "sky", "shape", "surf", "thunder", "violet", "water", "wildflower", "wave", "water", "resonance", "sun", "wood", "dream", "cherry", "tree", "fog", "frost", "voice", "paper", "frog", "smoke", "star"};
return (
adjs[(int) Math.floor(Math.random() * adjs.length)] +
"_" +
nouns[(int) Math.floor(Math.random() * nouns.length)]
);
}
private String getRandomColor() {
Random r = new Random();
StringBuffer sb = new StringBuffer("#");
while(sb.length() < 7){
sb.append(Integer.toHexString(r.nextInt()));
}
return sb.toString().substring(0, 7);
}
}
The MemberData
class is super minimal and will later be serialized into JSON and sent to users by Scaledrone.
class MemberData {
private String name;
private String color;
public MemberData(String name, String color) {
this.name = name;
this.color = color;
}
// Add an empty constructor so we can later parse JSON into MemberData using Jackson
public MemberData() {
}
public String getName() {
return name;
}
public String getColor() {
return color;
}
}
Sending messages
To send (or publish) the message to the Scaledrone room we need to add a onClick()
handler to the ImageButton
in the activity_main.xml
file.
<ImageButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:scaleType="fitCenter"
android:padding="20dp"
android:layout_marginHorizontal="10dp"
android:background="@drawable/ic_send_black_24dp"
android:onClick="sendMessage"/> <!-- 👈 This is the onClick that we just added -->
Let's add the sendMessage()
function to the MainActivity
. If the user has input something we send the message to the same observable-room as we subscribed to above. After the message has been sent we can clear the EditText
view for convenience.
Scaledrone will take care of the message and deliver it to everyone that has subscribed to the observable-room room in your channel.
public void sendMessage(View view) {
String message = editText.getText().toString();
if (message.length() > 0) {
scaledrone.publish("observable-room", message);
editText.getText().clear();
}
}
Displaying messages
As seen in the layout file the messages are going to be displayed via ListView
. To use a ListView
you need to create a class that extends android.widget.BaseAdapter
. This class is then used as the state of the ListView
.
Let's define our MessageAdapter
as well as the Message
class itself. The Message
class will hold all the needed info to render a single message.
// Message.java
public class Message {
private String text; // message body
private MemberData memberData; // data of the user that sent this message
private boolean belongsToCurrentUser; // is this message sent by us?
public Message(String text, MemberData memberData, boolean belongsToCurrentUser) {
this.text = text;
this.memberData = memberData;
this.belongsToCurrentUser = belongsToCurrentUser;
}
public String getText() {
return text;
}
public MemberData getMemberData() {
return memberData;
}
public boolean isBelongsToCurrentUser() {
return belongsToCurrentUser;
}
}
The MessageAdapter
defines how we render our rows within the ListView
.
// MessageAdapter.java
public class MessageAdapter extends BaseAdapter {
List<Message> messages = new ArrayList<Message>();
Context context;
public MessageAdapter(Context context) {
this.context = context;
}
public void add(Message message) {
this.messages.add(message);
notifyDataSetChanged(); // to render the list we need to notify
}
@Override
public int getCount() {
return messages.size();
}
@Override
public Object getItem(int i) {
return messages.get(i);
}
@Override
public long getItemId(int i) {
return i;
}
// This is the backbone of the class, it handles the creation of single ListView row (chat bubble)
@Override
public View getView(int i, View convertView, ViewGroup viewGroup) {
MessageViewHolder holder = new MessageViewHolder();
LayoutInflater messageInflater = (LayoutInflater) context.getSystemService(Activity.LAYOUT_INFLATER_SERVICE);
Message message = messages.get(i);
if (message.isBelongsToCurrentUser()) { // this message was sent by us so let's create a basic chat bubble on the right
convertView = messageInflater.inflate(R.layout.my_message, null);
holder.messageBody = (TextView) convertView.findViewById(R.id.message_body);
convertView.setTag(holder);
holder.messageBody.setText(message.getText());
} else { // this message was sent by someone else so let's create an advanced chat bubble on the left
convertView = messageInflater.inflate(R.layout.their_message, null);
holder.avatar = (View) convertView.findViewById(R.id.avatar);
holder.name = (TextView) convertView.findViewById(R.id.name);
holder.messageBody = (TextView) convertView.findViewById(R.id.message_body);
convertView.setTag(holder);
holder.name.setText(message.getMemberData().getName());
holder.messageBody.setText(message.getText());
GradientDrawable drawable = (GradientDrawable) holder.avatar.getBackground();
drawable.setColor(Color.parseColor(message.getMemberData().getColor()));
}
return convertView;
}
}
class MessageViewHolder {
public View avatar;
public TextView name;
public TextView messageBody;
}
Receiving messages
Now that we can display and render our chat bubbles we need to hook up the incoming messages with the MessageAdapter
that we just created. We can do that by going back to the MainActivity
class and finishing the onMessage()
method.
Scaledrone uses the popular Jackson JSON library for serializing and parsing the messages, and it comes bundled with the Scaledrone API client. Please see the Jackson docs for best practices on how to parse the incoming Scaledrone messages and users data.
@Override
public void onMessage(Room room, final JsonNode json, final Member member) {
// To transform the raw JsonNode into a POJO we can use an ObjectMapper
final ObjectMapper mapper = new ObjectMapper();
try {
// member.clientData is a MemberData object, let's parse it as such
final MemberData data = mapper.treeToValue(receivedMessage.getMember().getClientData(), MemberData.class);
// if the clientID of the message sender is the same as our's it was sent by us
boolean belongsToCurrentUser = receivedMessage.getClientID().equals(scaledrone.getClientID());
// since the message body is a simple string in our case we can use json.asText() to parse it as such
// if it was instead an object we could use a similar pattern to data parsing
final Message message = new Message(receivedMessage.getData().asText(), data, belongsToCurrentUser);
runOnUiThread(new Runnable() {
@Override
public void run() {
messageAdapter.add(message);
// scroll the ListView to the last added element
messagesView.setSelection(messagesView.getCount() - 1);
}
});
} catch (JsonProcessingException e) {
e.printStackTrace();
}
}
And we're done!
Hopefully, this tutorial helped you build your very own chat app. You can find the full source code or run the working prototype on GitHub. If you have any questions or feedback feel free to contact us.
This tutorial only scratched what Scaledrone can do for you and is the ideal basis for any of your future realtime needs.
Looking to build the same app for iOS using Swift? Check out our iOS chat tutorial.
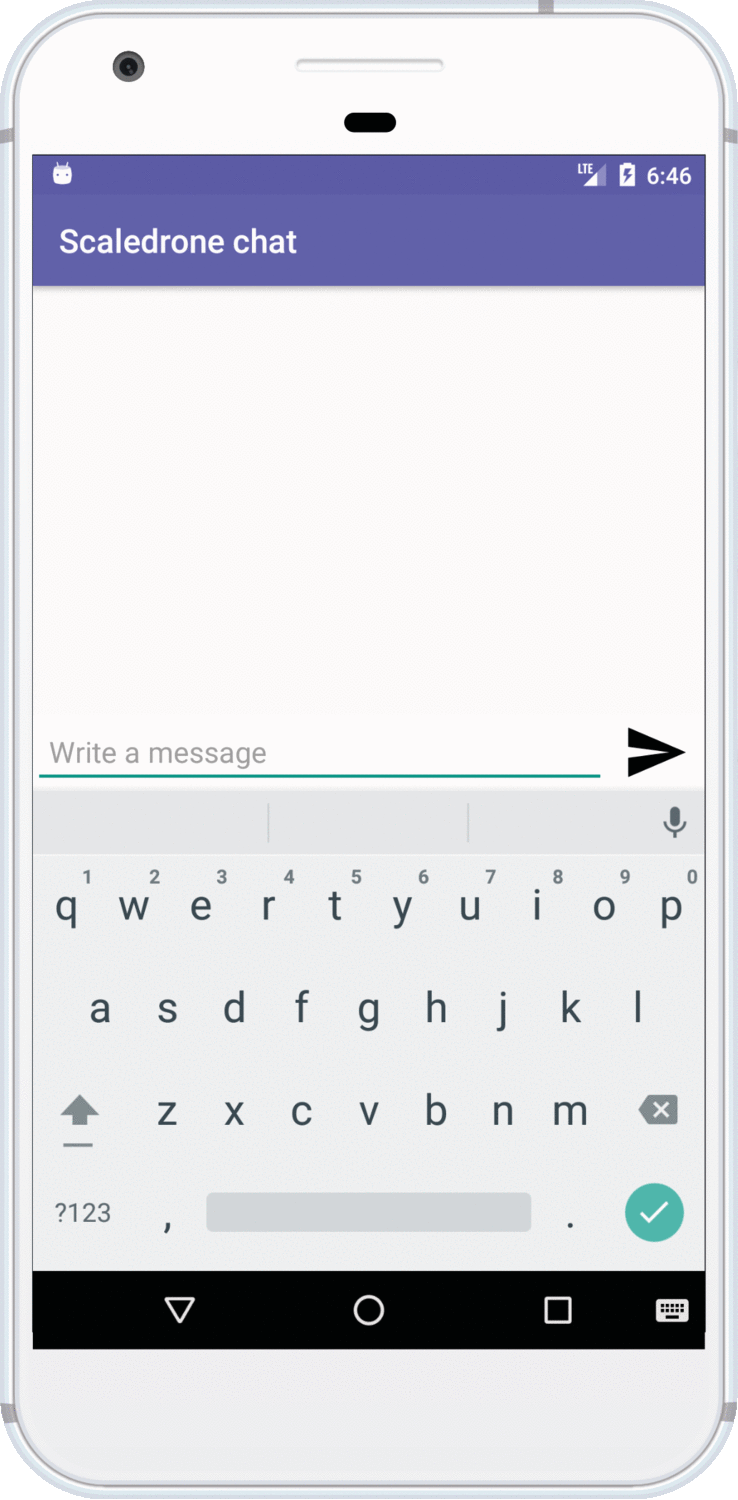
Last updated July 1st 2023